Game Dev Reference #2: Building a Snake Game in Python
Last time, I had built a Tetris game in Python, and now I’m back with another classic… Snake. The movement turned out kind of funny, so make sure to check it out after reading this blog. I included the link at the end of this. This was just my second time making a game, so I know there can be lots of improvements. If you have any suggestions to make the animation look better or add something like power-ups, comment them below and I’ll update the code.
Sup gamers, Sloth here, and if you haven’t guessed already, today I’m gonna talk about my experience making a snake game in Python. So, a week after my Tetris blog, Reaper was back with another project for me. At first, he told me to think of a game and I chose a text RPG. You know, like the Epic RPG in Discord. Of course, mine would focus more on different abilities and weapon types and all, so I figured it will go more than a thousand lines. I think Reaper realized I was gonna procrastinate like anything during this. Why else would he tell me to make something incredibly easy like Snake?… Well, that was what I’d thought before I found it was more complicated than I’d given it credit for.
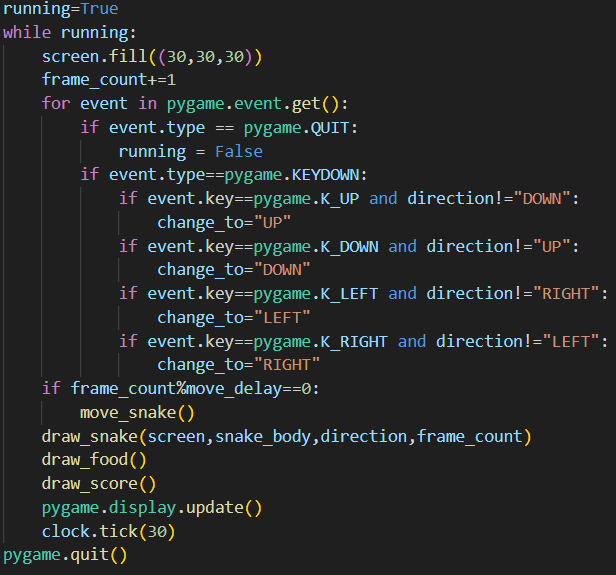
Let’s start with the logic first. I would need to create a game window, then display the snake and food. Of course, the snake can be a line of blocks and the food a circle. I would have to update the screen continuously so the snake moves in the direction of the last key input without stopping. The directions would be left, right, up and down. I also needed to make sure that the snake couldn’t turn right when it was moving left and such. I could store the body positions of the snake in a tuple list. Then, I gotta make the tail and the rest of the body follow the head. That should do it for the movement. Next, I should grow a new segment at its tail whenever it collides with the food. Such collisions should also make the food respawn randomly in any part of the screen that is not inhabited by the snake’s body. Oh, and the score will increase by ten.
Now, there are two versions of the classic snake game. One is where if the snake hits a border of the screen, it dies. It seemed kinda basic, so I went with the other one. That is, if the snake collides with one side of the border, it comes out of the opposite side. So, if the snake hits the left side of the screen, it’s head and then the rest of its body appear to go inside the left end and come out of the right. As if there was a portal or something. Next, I had to give it haphephobia or the fear of being touched, sometimes even by oneself (yeah, I googled it while writing this). Basically, the game restarts when it touches itself. Technically, this keeps the game running infinitely, unless you close the window. Basically, it translates to me being too lazy to write a restart window. By the time the reel will be out, I’ll have added one though.
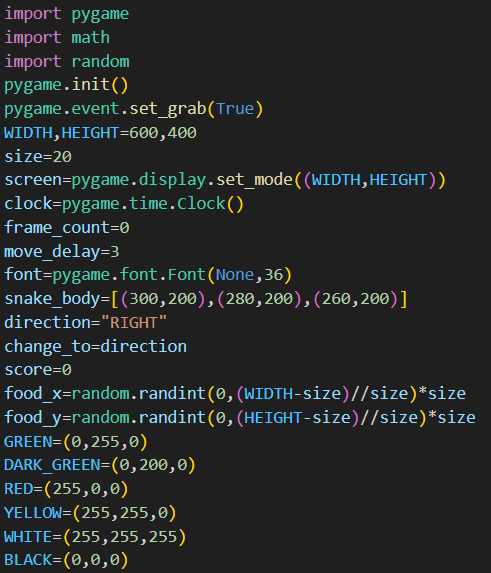
The modules I needed were pygame (for creating the screen, detecting key presses, changing the screen after a certain time period), math (for adding a slight curve to the movement, since nobody likes a snake that moves straight) and random (for the food). Since I started making games (I know I made only two, but hear me out), I’ve been really impressed by pygame. It has so many options, you can customize it in so many different ways. But of course, I know big 3D games can’t be designed with it. Such a shame! First, I set up the game window. I made it 600 by 400 pixels, with each block having 20 pixels. I set up the clock and fps to ten. Remember this, for this was my major mistake.
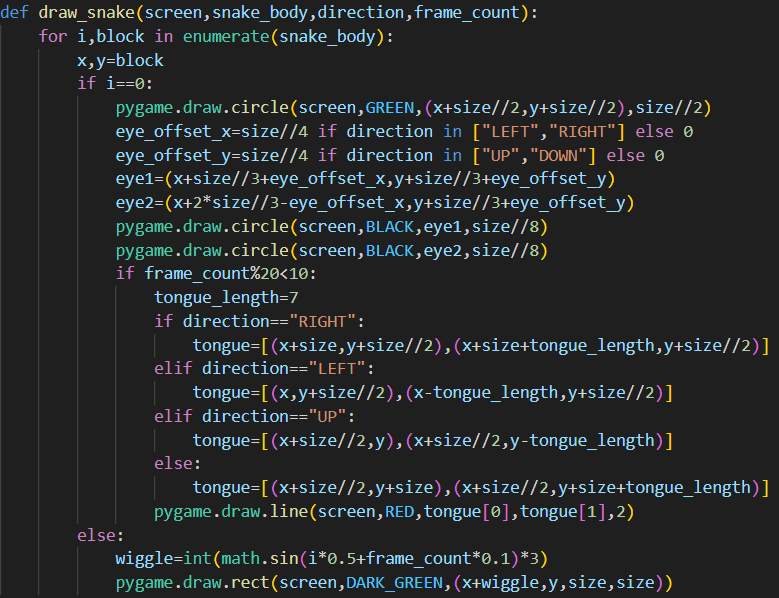
Then, I drew the snake. It had a circular green head, two black eyes, a tongue that flickered every few seconds and three blocks at the beginning. The first mistake I made was I had drawn the tongue outside the circular head using pygame.draw.line(), and since pygame.draw.circle() doesn’t clear pixels, this made the head look like a square when the tongue flickered. I changed the code accordingly, and I could see the snake of three blocks standing idly. Not gonna lie, I felt like a god, creating a creature, giving movement to it. It was the same as when I would create new worlds in my stories. I guess, that’s why it’s called development.
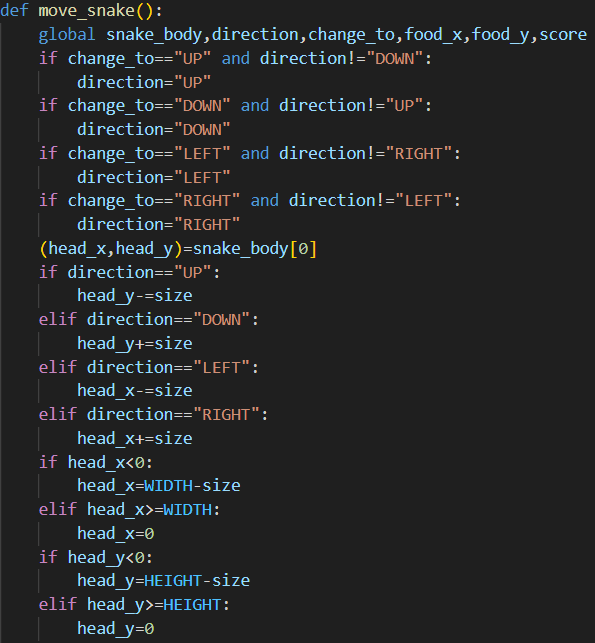
For the movement, I added a variable called direction, and then I added another one called change_to. This was for storing the direction the snake would move when an arrow key was pressed. Then I added some conditions which prevented the snake from going reverse of how it had been moving previously. The way I gave it movement was, I added a new snake head at the next box after each frame then moved the rest of the body in that direction. Like this, I made the movement continuous.
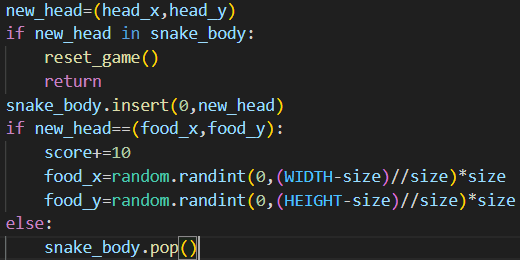
Next was food, and the snake’s growth. The food was an yellow circle (not creative, I know). The logic here was that if the coordinates of the snake’s head matched the coordinates of the food, the food disappeared and the program added another green square to the snake’s body along with its forward movement. Or that’s how it seemed. See, the way the movement worked, every frame, the head would be replaced by a new head next to it in the direction of the snake. Then, the previous head will be replaced by a green square and we’ll check if the snake has eaten the food. If not, we remove the last segment of its body, and it will appear to have moved one square forward. Genius, I know.
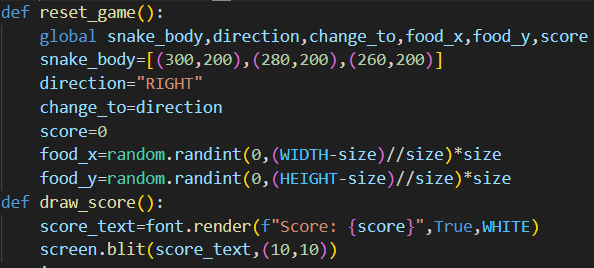
Lastly, I added the collision system to end the game when it bites itself. I also added a score display. So far, so good. But when I ran the program, the snake just kept on moving right without registering any key input. Now, after some tinkering with code, I discovered the mistake was with the fps. Remember I had set it to ten? I increased it to thirty to make it worked. But that created another problem. The snake was moving too fast. So, I added a delay system to make the snake move every three frames. And there you have it, an infinitely running snake game.
Now, I have added the code to GitHub too so you can copy it and run it in your computers by clicking here. Also, don’t forget to comment if you’d like to see any new games in the future. Moral of the blog? My second game, and I’m feeling more confident than ever. So long, peeps. Happy procrastinating!
Discover more from Ge-erdy Verse
Subscribe to get the latest posts sent to your email.