Game Development Journey #1: My First Steps, Designing a Simple Tetris Game in Python
Say, have you guys missed me? I haven’t been posting as many blogs as in the past. I do have a good reason (excuse). I’ve been interested in coding recently. Why? Well, I want to become a… game developer! Shocking, right? So, anyway, this blog is the start of a new series, one where I record how I slowly enter the game dev world AND MAKE IT MINE, but let’s not get ahead of ourselves.
Sup gamers, Sloth here, and if you haven’t guessed already, today I’ll be partly explaining and partly advising how to not procrastinate, but fully bragging about my newly-learnt skill: Coding. The last two months or so, I’ve been coding now and then, so Reaper gave me a project out of nowhere. Make a basic game in Python. Now, you may know about game development. It involves creating a game from scratch and maintaining it. Now there are many stages to it. You first come up with a game plot, the characters, how it will be played, and more. Then, you create the game environments, characters and other visual assets. These are usually done by game engines and 3D software. After that, comes sound. This includes background music, character dialogues, fighting noises, if any, and others. Next, you write the code that brings all these together and create something that’s worthy of being called a game. Of course, you need to test your game and ensure its free of bugs and glitches. Finally, you release it to the platforms you want, and start promoting it.
So, as you can see, it’s a lot of work. Was I doing all this? Nah, I was said to create a game of Tetris, nothing else. And if you follow our YouTube and Instagram accounts, you’ve already seen how fast I was typing. Since this was a personal project, I didn’t give any comments or gaps, and the code was 122 lines. And it took me around 2 hours, heh. My genius is frightening.
Let’s start from the beginning. The coding I was doing was not related to game development so I had to research and learn many things. Here’s the way I thought of solving the problem. First, I create a game board to display the tetrominoes. I create a grid, where each cell will either be empty or contain a block. This information can be stored in a 2D grid, with 0’s corresponding to empty cells and 1’s to filled ones. Then, I design the tetrominoes. This will be easy as the shapes only have like four blocks each. There are seven shapes: I, O, T, L, J, S and Z, and their rotation can be handled by 2D matrices.
Next comes the movement. I need to move down the pieces every few milliseconds. They should also be able to move horizontally and rotate, provided they don’t collide. Which brings us to another important aspect. I need to check if a piece hits the bottom, overlaps with another block, or goes outside the grid. Also, if a piece hits the floor, or if there’s another piece under it, we need to lock it in place. And if a row is filled, it gets cleared and everything below it shifts down. The game-over condition will be if a new piece spawns and immediately collides with another piece.
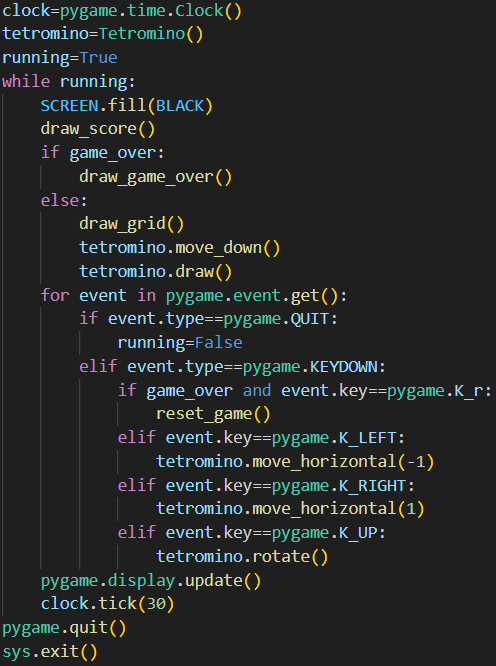
I figured I needed four modules for this: pygame (for graphics, input handling and the game loop), random (for piece selection), time (for controlling the game speed), and sys (for smoothly exiting). So, I started by creating a 600×600 window for the game, filled it with black, and ran it at 30 fps so that your potato PC could run it. Next, I created a 20×20 grid, each cell being 30×30 pixels and bordered by grey lines. Yes, I made it square instead of a rectangle. Not only does this add a unique touch, but it also makes the game harder, befitting of a soulsborne player. I used the pygame.draw.rect() command to draw it. I stored this in a function draw_grid().

Then, I defined seven tetromino shapes as lists, assigned colours to them, and stored them in a dictionary for easy access. With that out of the way, I decided to spawn a random tetromino at the top of the grid. For that, I created a class Tetromino that creates a new piece with a random shape and colour. I defined a constructor to initialize a piece when it is created. It picks a random shape, gets the matrix for it, picks the colour and stores its position. And, I made a draw() function to draw the shape on the screen. It loops through the shape’s matrix and draws a solid rectangle wherever it encounters a 1. Next, I had to add movement. So, I added a timer and used pygame.time.get_ticks() to control speed.
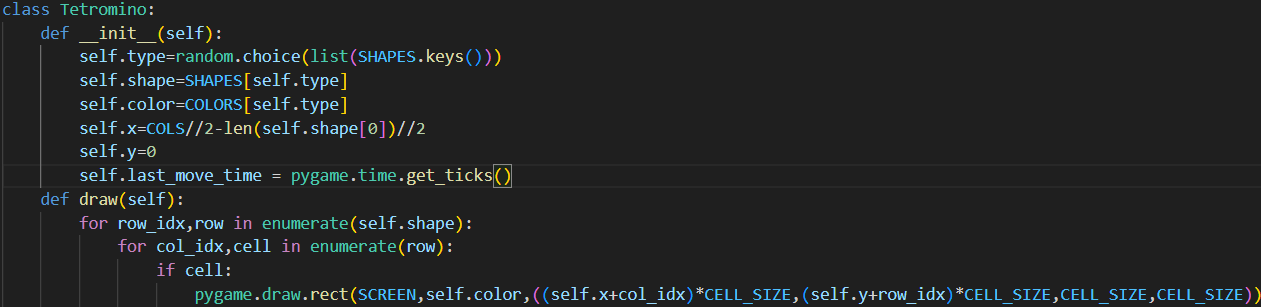
I also added a move_down() function to control the vertical movement. The logic behind this was updating the y coordinate. Then, I added a move_horizontal() function that helped you move a shape left and right. To prevent it from going outside the grid, I used if 0<=new_x<=COLS-len(self.shape[0]). And, pygame.KEYDOWN() detected arrow presses.
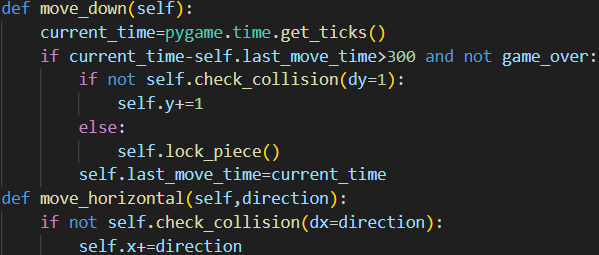
Next, I added a rotate() function for rotating the pieces whenever I pressed the up arrow key. I used zip(*matrix[::-1]) to rotate the matrix.

Then, I added a grid to track placed blocks and added a check_collision() function to make sure blocks don’t overlap. I also updated the move_down() and rotate() functions to check for collisions, and created lock_piece() to lock the pieces in place as they reach the bottom. After that, it was time for clearing full rows, so I added clear_rows() to clear filled rows and move the blocks above it down by one space. Then, I inserted those cleared rows at the top.
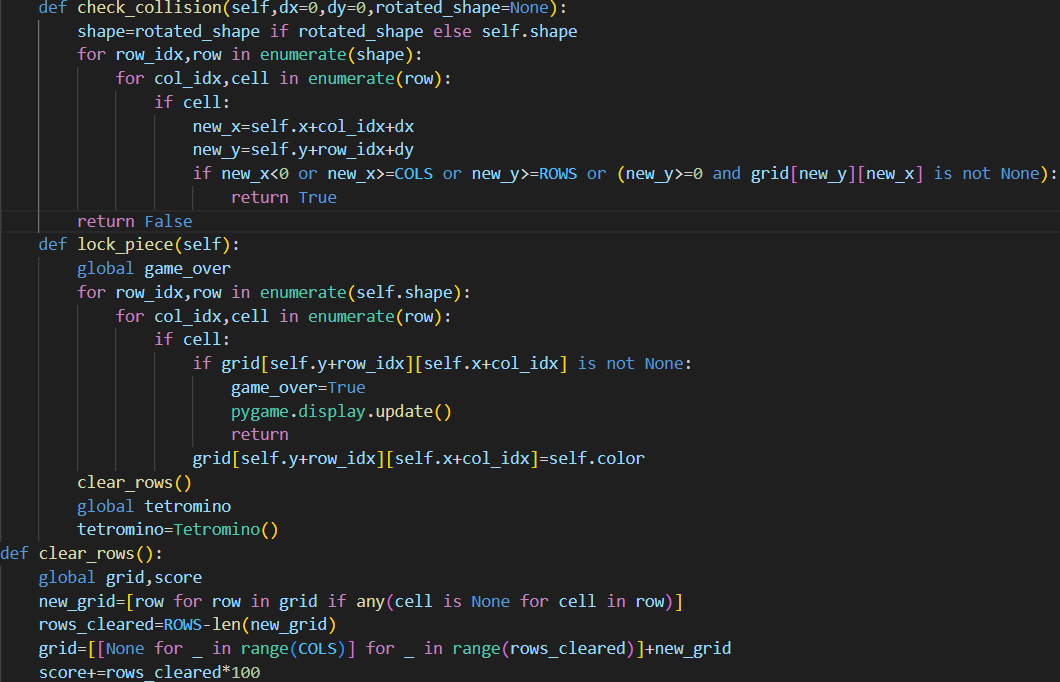
I also added a score variable and a draw_score() to display it with the blit() command. Finally, I added a game over screen that stops the loop and displays when a piece gets locked at the top. After that, you can press R to play again.
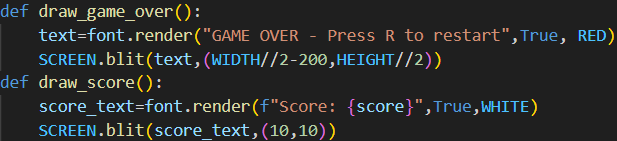
That was the breakdown of the code I’ve written. I couldn’t explain all the lines, or the blog would be too long and… let’s face it, boring. I’ll upload the program to GitHub so that you can download it by clicking here. Have fun playing it, coz it’s harder than your typical Tetris game. Moral of the blog? THIS IS JUST THE BEGINNING. I’LL TAKE OVER THE GAMING INDUSTRY ONE DAY. So long, peeps. Happy procrastinating!
Discover more from Ge-erdy Verse
Subscribe to get the latest posts sent to your email.
Gaming as Therapy: How Video Games Improve Mental Health with Real Data - Ge-erdy verse
February 16, 2025 @ 1:47 pm
[…] thought a classic puzzle game could be your mental health MVP? Enter Tetris. Recent buzz on TikTok suggests that playing Tetris can help cope with trauma. Researchers, like […]